728x90
문자열 입출력하기
#define _CRT_SECURE_NO_WARNINGS
#include <stdio.h>
int main()
{
char fruit_name; //stores only one character
printf("what is your fruit? \n");
scanf("%c", &fruit_name); // &
printf("You like %c \n", fruit_name);
return 0;
}
다음과 같이 문자열 한 글자
를 입력받을 수 있다.
여러 문자열을 입력받고 싶다면 아래와 같이 입력하면 된다.
#define _CRT_SECURE_NO_WARNINGS
#include <stdio.h>
int main()
{
char fruit_name[40]; //stores only one character
printf("what is your fruit? \n");
scanf("%s", fruit_name); // &
printf("You like %s \n", fruit_name);
return 0;
}
달라진 점은 다음과 같다.
- [40]과 같이 문자열 수를 할당해 배열로 바꾼다.
- %s로 바꿔준다.
- &를 없애준다!!
&를 없애줄 수 있는 이유는,fruit_name[40]
자체가 문자열을 접근하는메모리 주소 자체
를 나타내기 때문에 &가 필요없기 때문이다.
sizeof 연산자
#include <stdio.h>
#include <stdlib.h> //malloc()
struct Mystruct //4에서 사용
{
int i;
float f;
};
int main()
{
/* 1. size of basic types*/
int a = 0;
unsigned int int_size1 = sizeof a;
unsigned int int_size2 = sizeof(int);
unsigned int int_size3 = sizeof(a);
size_t int_size4 = sizeof(a);
size_t float_size = sizeof(float);
printf("size of int = %u bytes \n", int_size1);
printf("size of int = %zu bytes \n", int_size4);
printf("size of float type = %zu bytes\n\n", float_size);
/* 2. sizeof arrays*/
int int_arr[30]; //int_arr[0]=1024;
int* int_ptr = NULL; //pointer: 추후 설명
int_ptr = (int*)malloc(sizeof(int) * 30);
printf("size of array = %zu bytes \n", sizeof(int_arr));
printf("size of pointer = %zu bytes \n", sizeof(int_ptr));
/* 3. sizeof character array*/
char c = 'a';
char string[10]; //maximally 9 char + '/0' (null character)
size_t char_size = sizeof(char);
size_t str_size = sizeof(string);
printf("size of char type = %zu bytes \n", char_size);
printf("size of string type = %zu bytes \n", str_size);
/* 4. size of structure */
printf("%zu \n", sizeof(struct Mystruct)); // 4bytes + 4bytes
return 0;
}
배열, 동적 할당을 받는 메모리, string 등등 각각 인지하자.(추후에 자세히 설명)
문자열이 메모리에 저장되는 구조
int main()
{
int a = 1;
int int_arr[10] = { 0,1,2,3,4,5,6,7,8,9 };
printf("%i %i %i \n", int_arr[0], int_arr[1], int_arr[9]);
char c = 'a';
char str1[10] = "hello";
char str2[10] = { 'H', 'i' };
printf("%c\n", c);
printf("%s\n", str1);
printf("%s\n", str2); //array 중 0을 만나면 printf 내부에서 종료함!
printf("%hhi %hhi %hhi %hhi %hhi \n",
str2[0], str2[1], str2[2], str2[3], str2[4]); //0도 출력하게 test
//char str3[10] = "hello,world"; //array size is not enough
char str3[20] = "hello, world"; //array size is enough
printf("%s\n", str3);
char str4[20] = "hello, \0world"; //"\0" can stop printf!
printf("%s\n", str4);
}
strlen() 함수
#include <string.h> //strlen and more
int main()
{
char str1[100] = "hello";
char str2[] = "hello";
char str3[100] = "\0"; //빈칸과 \0(null)은 다르다!
char str4[100] = "\n";
printf("size: %zu len: %zu \n", sizeof(str1), strlen(str1));
printf("size: %zu len: %zu \n", sizeof(str2), strlen(str2));
printf("size: %zu len: %zu \n", sizeof(str3), strlen(str3));
printf("size: %zu len: %zu \n", sizeof(str4), strlen(str4));
/* Extra */
char *str5 = (char*)malloc(sizeof(char) * 100);
str5[0] = 'H'; str5[1] = 'e'; str5[2] = 'l'; str5[3] = 'l'; str5[4] = 'o';
str5[5] = '\0';
printf("%zu %zu \n", sizeof(str5), strlen(str5));
return 0;
}
기호적 상수와 전처리기 #define
3.141592 PI와 같이, 한 번 define 해두면 계속 편리하게 사용할 수 있는 symbolic constants를 사용하자.
주로 대문자로 define 해준다!
#define PI 3.141592f
#define AI_NAME "Jarvis"
int main()
{
float radius, area;
printf("I'm %s \n", AI_NAME);
printf("please input radius \n");
scanf("%f", &radius);
area = PI * radius * radius;
printf("area is %f \n", area);
return 0;
}
명백한 상수들(Manifest Constants)
- Manifest Constants: A value that is assigned to a symbolic name at the beginning of a computer program and is not subject to change during execution.
- Mainly uses
limits.h
andfloat.h
#include <limits.h> //INT_MAX, ... , etc
# include <float.h> //FLT_MAX, ..., etc
printf() 함수의 변환 지정자들
printf(제어-문자열, 아이템1, 아이템2, ...)
int main()
{
double d = 3.14159265358979323846264388327950; //가능한 메모리만큼 알아서 짤림
printf("%c\n", 'A'); //글자 하나만 표현할때는 작은 따옴표!
printf("%s", "i love you\n");
printf("Even if there's a small chance, \
we owe this to everyone. \n");
printf("\n");
printf("%d %i %i %i \n", 1004, 1234, INT_MAX, UINT_MAX); //UINT_MAX overflow로 -1 출력
printf("%u %u %u \n", 1024, -1, UINT_MAX); // -1을 넣어서 overflow 발생
printf("\n");
printf("%f %f %lf\n", 3.141592f, d, d); //l in lf is ignored
printf("%a %A\n", d, d);
printf("%e %E\n", d, d);
printf("\n");
printf("%g %g\n", 123456.789, 1234567.89); //%g 는 자동으로 %f, %e 중 골라서 출력함
printf("%G %G\n", 123456.789, 1234567.89);
printf("%g %g\n", 0.00012345, 0.000012345);
printf("%G %G\n", 0.00012345, 0.000012345);
printf("\n");
printf("%o\n", 9);
printf("%p\n", &d); // pointer of operator // d변수가 사용하는 memory address 출력
printf("\n");
printf("%x %X\n", 11, 11); //소, 대문자로 16진수 출력
printf("%% \n", d); // Note the warning. d is ignoreds
변환 지정자의 수식어들
형식 지정자
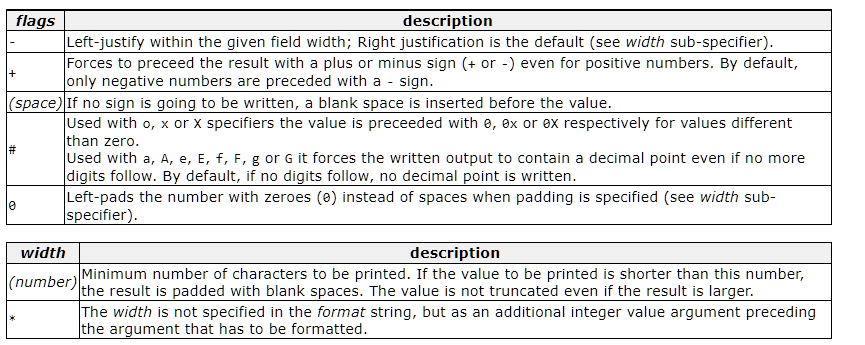
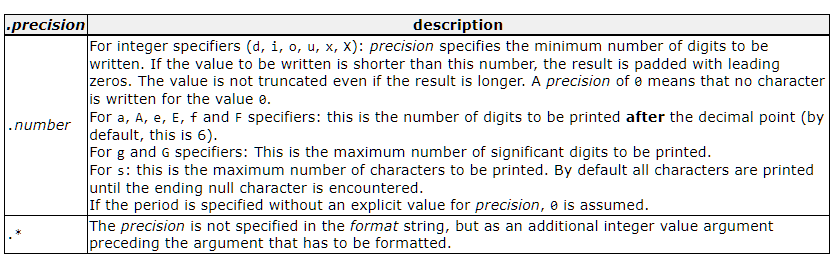
int main()
{
printf("%10i\n",1234567 );
printf("%-10i\n",1234567 );
printf("%+i %+i\n", 123, -123);
printf("%i %+i\n", 123, -123);
printf("%X\n", 17);
printf("%#X\n",17 );
printf("%05i\n", 123); //숫자앞에 0을 붙이면 0으로 빈칸을 채운다.
printf("%*i\n", 7, 456); //7이 *자리에 들어가게 된다!
printf("\n ---------Precision--------- \n");
printf("%.3d\n", 1024);
printf("%.5d\n", 1024);
printf("%.3f\n", 123.1234567);
printf("%10.3f\n", 123.1234);
printf("%010.3f\n", 123.1234);
printf("%.5s\n", "ABCDEFGHI");
printf("%.s\n", "ABCDEFGHI"); // %.만 넣으면 숫자 0이 들어간것과 동일하게 작동함
printf("\n ---------Lenght--------- \n");
printf("%hhd %hd %d\n", 257, 257, 257);
printf("%d %lld %lld\n", INT_MAX + 1, INT_MAX + 1, 2147483648LL); //x86, x64 결과가 다름!
return 0;
}
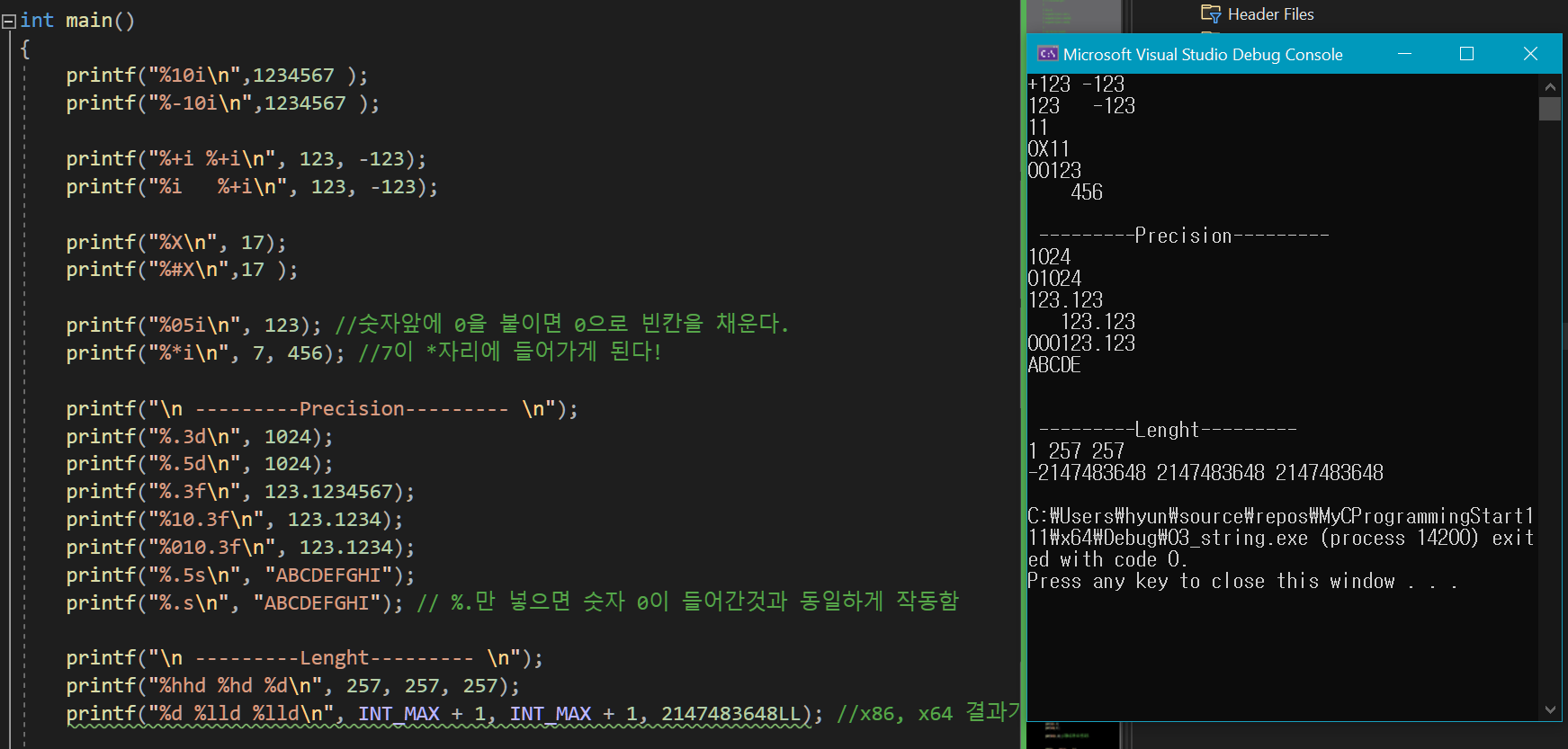
printf()함수가 인자들을 해석하는 과정과 주의사항
int main()
{
float n1 = 3.14;//4bytes
double n2 = 1.234; //8bytess
int n3 = 1024; //4bytes
printf("%f %f %d\n -------------- \n\n", n1, n2, n3);
//Note the warnings in output window
printf("%d %d %d \n\n", n1, n2, n3); // 4, 4, 4 (N, N, N)
printf("%lld %lld %d \n\n", n1, n2, n3); // 8, 8, 4 (N, N, Y)
printf("%f %d %d \n\n", n1, n2, n3); // 8, 4, 4 (Y, N, N)
printf("%f %lld %d \n\n", n1, n2, n3); // 8, 8, 4 (Y, N, Y)
}
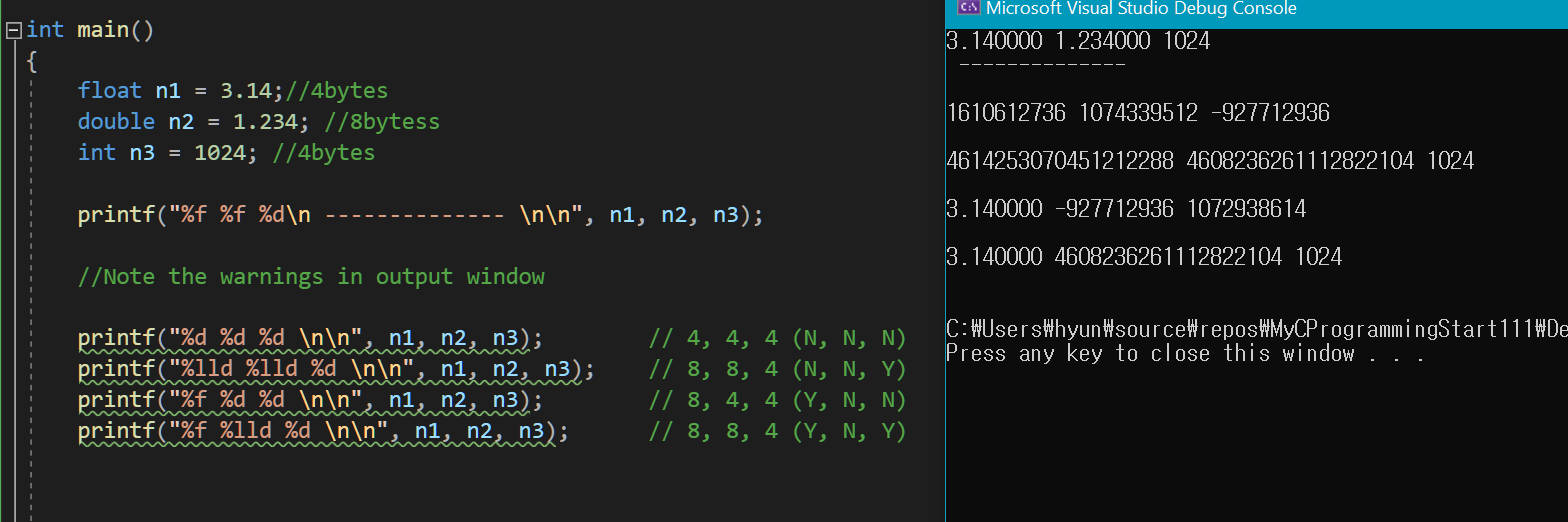
- float는 printf()에 입력으로 들어갈 때, double(8byte)로 받게 된다. 그렇기 때문에, 출력 형식도 8byte로 지정을 해 줘야 뒤쪽에 출력할 n2, n3로 문제 없게 출력된다.
scanf() 함수의 사용법
- 차이점, 주의사항 위주 설명
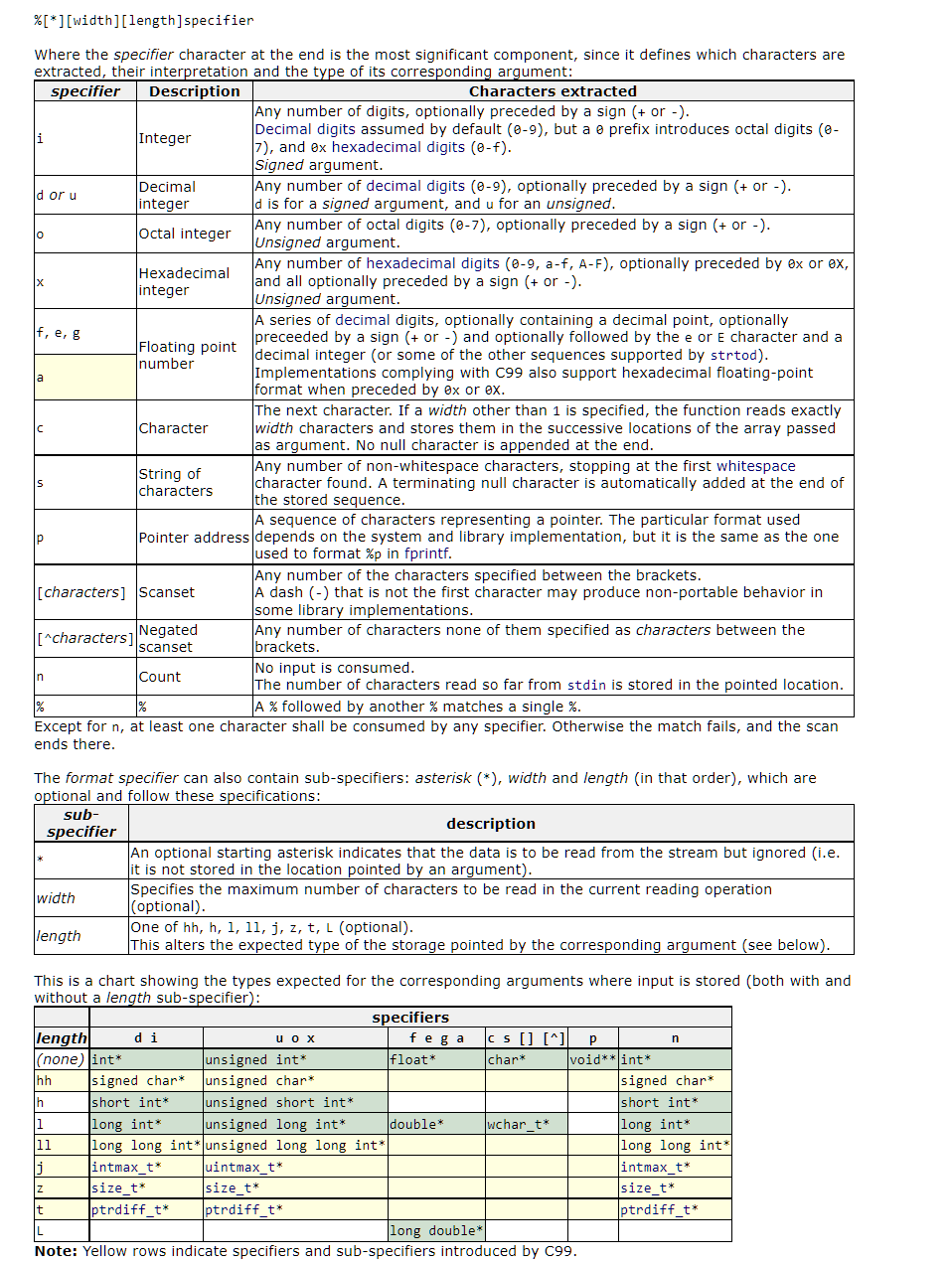
출처: https://www.cplusplus.com/reference/cstdio/scanf/
/* mutliple inputs with blank separators*/
int i;
float;
char str[30];
scnaf("%d %f %s\n", &i, &f, str); //note % is absent in front of str!
printf("%d %f %s\n", i, f, str);
/* unsigned as unsigned*/
unsigned i2;
scanf("%u", &i2); //try negative number
printf("%u\n", i2);
/* floating point numbers*/
// l for double for %f, %e, %E, %g
double d = 0.0; //CAUTION: printf와 float가 대응된다고 생각하지 말기
scanf("%lf", &d); //lf, try E notation //lf or f? Try!
printf("%f\n", d); // f
/* width*/
char str[30];
scanf("%5s", str); //width
printf("%s \n", str);
/* h modifier*/
char i;
scanf("%hhd", &i); //char type!!(signed char -128~+127) //try large numbers
printf("%i \n", i);
/* integer with characters*/
int i;
scanf("%i", &i); //try 123ab, 123a456
printf("%i\n", i);
/* j modifier */
intmax_t i; //long long int_max 제공
scanf("%ji", &i); //j는 int_max 받아들이는 의미로 붙임
printf("%ji", i);
/* regular characters */
int a, b;
scnaf("%d, %d", &a, &b);
printf("%d %d \n", a, b);
/* char receives blank*/
int a, b;
char c;
scanf("%d%c%d", &a, &c, &b); //try 123 456 (blank)
printf("%d|%c|%d", a, c, b); // |: separator
/* return value of scanf */
int a, b;
int i = scanf("%d %d", &a, &b); // return value: item을 몇 개나 받았는지
printf("%d", i); //2!
/* modifier for printf() */ㅉ
int i = 123;
int width = 5; //from script file, scanf, etc.
printf("input width:");
scanf("%d", &width); //width를 지정할 수 있게
printf("%*d\n", width, i);
728x90
'Computer Science > 따라하며 배우는 C' 카테고리의 다른 글
07 If문 (0) | 2022.03.31 |
---|---|
06 For (0) | 2022.03.31 |
04 연산자 (0) | 2022.03.11 |
02 데이터와 C언어 (0) | 2022.03.03 |
01 C 언어를 소개합니다. (0) | 2022.03.03 |