728x90
변수와 상수
int angel = 1004;
- 변수: 어떤 값이 저장될 수 있는 메모리 공간
- 리터럴 상수(literal constant): 문자 그대로의 의미를 갖고 값이 변하지 않는 수
scanf() 함수의 기본적인 사용법
#include <stdio.h>
int main()
{
int i = 0;
scanf("%d", &i); // & : ampersand
printf("value is %d \n", i);
return 0;
}
이렇게 코드를 짠 후 build를 하면 오류가 나온다.
이를 해결하기 위해서는 2가지 방법이 있다.
- 코드 제일 위에
#define _CRT_SECURE_NO_WARNINGS
삽입 - project - properties - C/C+ - preprocessor에
define _CRT_SECURE_NO_WARNINGS
삽입
❓
&
를 붙여야 하는 이유??❗
&
를 붙이게 되면 변수의주소
를 넘겨주게 된다! 그 주소에 해당하는 메모리 값을 넘겨준다.값
을 넘겨주게 되면, 이후에 코드들에 값이 덮이기 때문에 보안의 문제가 있다고 한다.
또한, 여러 개 입력을 받게 해줄 수 있다는 장점도 있다.
정수의 오버플로우
#include <stdio.h>
int main()
{
unsigned int i = 0; // 4byte가 나옴!ㄴ
printf("%u \n",sizeof(unsigned int)); //unsigned int //4
printf("%u", sizeof(i)); // 4
return 0;
}
변수를 지정할 때, 변수가 가질 수 있는 최대/최소값 사이에서 지정해주어야 한다. 그렇지 않으면 오버플로우가 발생한다.
#include <stdio.h>
#include <limits.h>
int main()
{
unsigned int i = 0b11111111111111111111111111111111; // 4byte(32bit)가 나옴!
unsigned int u = UINT_MAX;
printf("%u\n", i);
printf("%u\n", u);
printf("%d\n", i); // signed int로 잘못 지정해주는 경우: -1이 나옴
unsigned int u_max = UINT_MAX;
signed int i_max = INT_MAX;
printf("max of uint = %u\n", u_max); //4294967295
printf("max of int = %d\n", i_max); //2147483647
return 0;
}
이렇게 코드를 돌려보면, min, max 값이 다름을 확인할 수 있다.
다양한 정수형들
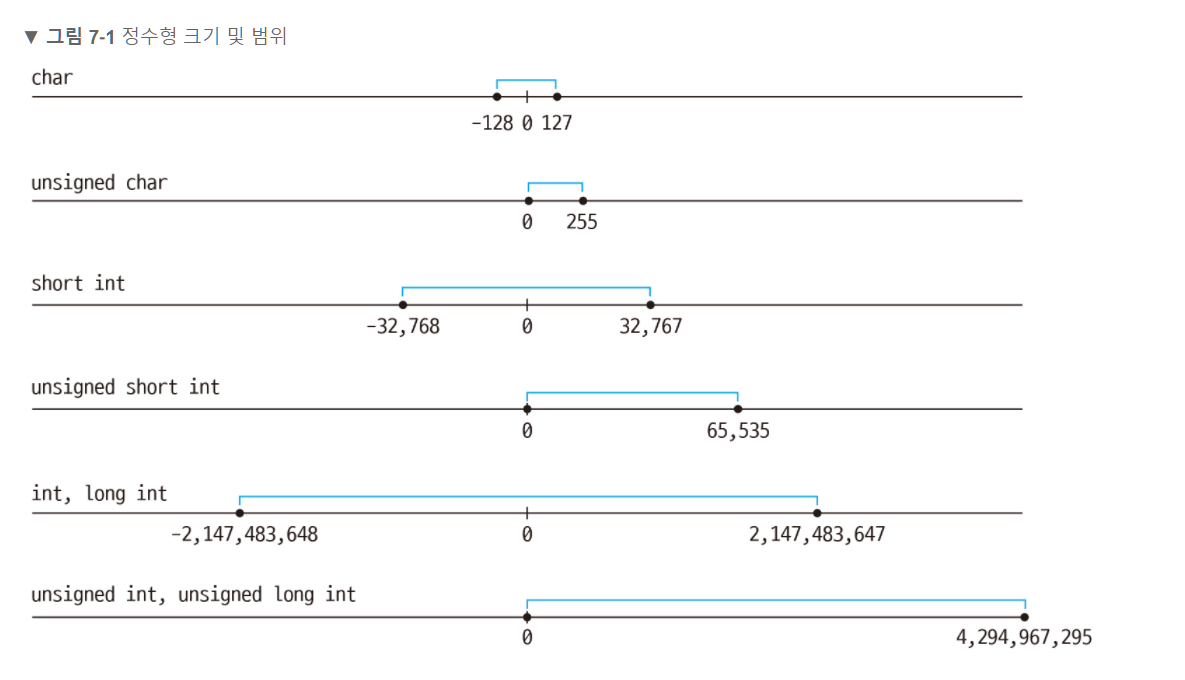
위와 같이, 여러 가지의 정수형 자료형이 있다.
이식성이 높은 고정 너비 정수형
변수, 자료형의 size를 정확하게 지정해줄 수 있다!
printf를 사용할 때, 형식 지정자를 모를 수도 있다. 그렇기에 <inttypes.h>
가 include되어있는 경우 type을 알아서 알 수 있다.(stdint.h는 없어도 된다.)
#include <stdio.h>
#include <stdint.h> //inttypes.h 있으면 없어도 됨
#include <inttypes.h>
int main()
{
int i;
int32_t i32;
int_least8_t i8;
int_fast8_t f8;
intmax_t imax;
uintmax_t uimax;
i32 = 1004;
printf("me32 = %d\n", i32);
printf("me32 = %" "d" "\n", i32);
printf("me32 = %" PRId32 "\n", i32); //PRId32: macro (`PRI`nt `d`ecimal `32`)
}
scanf()
를 사용할 때도 사용 가능하다!
문자형
컴퓨터는 문자들도 모두 숫자로 처리하여 인식한다. 그렇기에 ASCII chart를 통해 숫자로 변환하여 인식한다.
int main()
{
char c = 'A';
char d = 65; //d="A"
int salary= 100000;
printf("%c %hhd \n", c, c);
printf("%c %hdd \n", d, d);
printf("%c \n", c + 1); // B
// escape sequence example
char a = '\a';
// printf("%c", a); //ring bell sound
// printf("\07"); // 8진수 7 // ring bell sound
// printf("\x7"); // 16진수 7 // ring bell sound
// printf("\x23"); // output sharp
printf("$ ______ \b\b\b\b\b\b");
scanf("%d", &salary);
return 0;
}
부동소수점형(Floating point types)
Scientific Notations
과학, 공학 분야에서 천문학적이게 큰 숫자를 다루기 위해 사용함.
- m x $10^n$ 로 표현하며, m은 significand, n은 exponent로 부름
- 123.45 -> 12345 x $10^-2$ = 1.2345 x $10^+2$
Normalized significand: 1.xxxx * $2^n$
메모리 저장
이번엔 부동소수점이 메모리에 어떻게 저장되는지를 살펴보자.
int main()
{
printf("%u\n", sizeof(float)); //4
printf("%u\n", sizeof(double)); //8
printf("%u\n", sizeof(long double)); //8이 나온다! double과 동일 vscode에서는 12나옴
// 선언
float f = 123.456f;
double d = 123.456;
float f2 = 123.456; //메모리 오류:truncation from 'double' to 'float' 오류 발생: double이 float보다 메모리 2배 사용
double d2 = 123.456;
int i = 3;
float f3 = 3.0f; //3.0 실수 표현을 꼭 해줘야 함!
double d3 = 3.0; //3.0
float f4 = 1.234E10f; //scientific notation //e(소문자)도 됨
float f5 = 0x1.1p1;
double d5 = 1.0625e0;
printf("%f %F %e %E \n", f, f, f, f);
printf("%f %F %e %E \n", d, d, d, d);
printf("%a %A \n", f5, f5);
printf("%a %A \n", d5, d5);
return 0;
}
부동소수점의 한계
int main()
{
//round off erorss (ex1)
float a, b;
a = 1.0E20f + 1.0f;
b = a - 1.0E20f;
printf("%F\n", b); //1.0이 나와야 하지만, 0이 나온다!
}
- 너무 범위 차이가 큰 숫자끼리 연산을 하면 이렇게 값이 정확히 출력되지 않는다!!
- 부동소수점 메모리를 사용하는 구조의 차이에서 기인한 오류이다.
- 또한, 0.01을 계속 더한다고 해서 1을 만들 수 없다!! 0.9999로 출력되는 오류가 있다.
int main()
{
//overflow
float max = 3.402823466e+38F;
printf("%f\n", max);
max = max * 100.0f;
printf("%f\n", max); //inf error
//underflow
float min = 1.401298464e-45F;
printf("%e\n", min);
min = min / 100.0f; //subnormal: 부동소수점 형의 정밀도로 표현할 수 없이 작은 수라서 0으로 날아가버린 숫자를 의미함
printf("%e\n", min);
float f = 104.0f;
printf("%f\n", f);
f = f / 0.0f;
printf("%f\n", f);
return 0;
}
위와 같이 overflow, underflow가 발생하기도 한다.
불리언형(Bool Type)
#include <stdbool.h>
int main()
{
printf("%u Byte \n", sizeof(_Bool)); //1byte
_Bool b1, b2;
b1 = 0;
b2 = 1;
printf("%d %d \n", b1, b2);
return 0;
}
- Bool은 0 또는 1이다!!
- False가 아니면 True로 본다!
복소수형
복소수는 만들어 사용하는 게 보편적이다.
728x90
'Computer Science > 따라하며 배우는 C' 카테고리의 다른 글
07 If문 (0) | 2022.03.31 |
---|---|
06 For (0) | 2022.03.31 |
04 연산자 (0) | 2022.03.11 |
03 문자열과 형식 맞춘 입출력 (0) | 2022.03.03 |
01 C 언어를 소개합니다. (0) | 2022.03.03 |